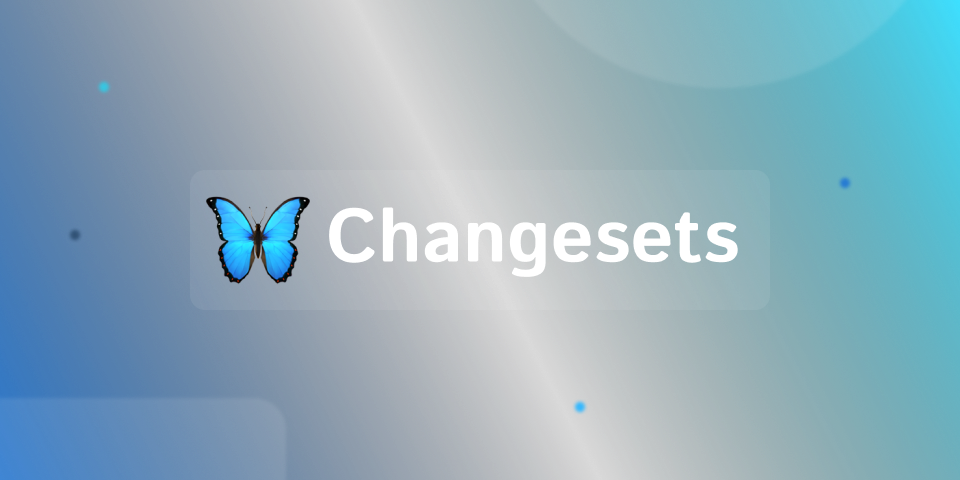
Effortlessly Publish Node.js TypeScript Packages with Changesets
By
D3OXY
By
D3OXY
Publishing and maintaining a Node.js package, especially one written in TypeScript, can be tricky. However, Changesets make version control and package publishing smooth and efficient. In this blog, you will learn how to use Changesets to streamline publishing your Node.js TypeScript npm packages. Along the way, we’ll provide practical code examples, tips, and references to official documentation.
Changesets are a way to manage version control and automate the publishing of npm packages. Whether you’re working with a monorepo or a single package, Changesets help by generating metadata that describes your changes. This allows you to automate your versioning and publishing workflow efficiently.
First, you’ll need to set up a basic Node.js and TypeScript project. Let’s walk through the steps:
Initialize the npm project:
npm init -y
Install TypeScript and other dependencies:
npm install typescript --save-dev
Create a basic tsconfig.json
:
{
"compilerOptions": {
"target": "ES6",
"module": "CommonJS",
"outDir": "./dist",
"rootDir": "./src",
"strict": true
}
}
Create your project files:
Let’s create a simple file src/index.ts
:
export const greet = (name: string): string => {
return `Hello, ${name}!`;
};
You now have a basic Node.js project with TypeScript ready to be published!
Changesets provide a streamlined way to handle versioning and publishing. Here’s how you can integrate Changesets into your project:
Install Changesets as a development dependency:
npm install @changesets/cli --save-dev
Initialize Changesets in your project:
npx changeset init
This will create a .changeset
folder with a config file. The configuration handles how Changesets work for your project.
When you make a change in your code, create a changeset to track it. A changeset describes what has changed, so the versioning system knows how to bump the package version.
Create a new changeset:
npx changeset
Follow the prompts to describe your change (whether it’s a major, minor, or patch update).
This will generate a .changeset
file like:
---
"my-package": patch
---
Fixed a bug in the greeting message.
This file is crucial for automating the versioning process later on.
Once you have a few changesets in place, you can bump versions and publish:
Version your package:
npx changeset version
This will automatically update the version in package.json
.
Build and publish your package:
npm run build
npm publish
If you’re using a CI/CD pipeline, you can automate this process to trigger whenever changes are merged into your main branch.
Here’s a script in your package.json
for using Changesets:
{
"scripts": {
"build": "tsc",
"release": "npx changeset version && npm publish",
"changeset": "npx changeset"
}
}
This script allows you to build, version, and publish your package in a single command:
npm run release
For more troubleshooting tips, refer to the official Changesets documentation.
Changesets offer a powerful and efficient way to manage versioning and package publishing. By following the steps outlined here, you’ll be able to streamline your workflow and publish your Node.js TypeScript packages with ease.