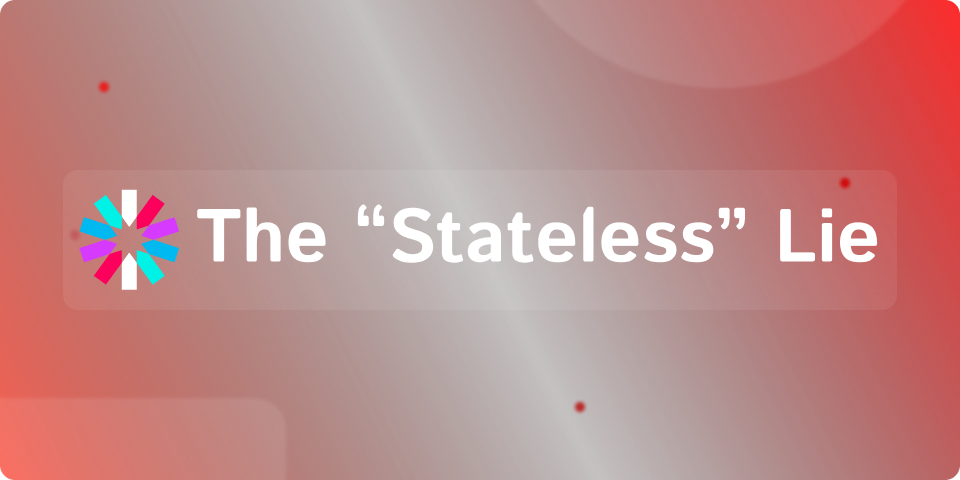
Stop Using JWT for Authentication: The Stateless Myth
By
D3OXY
By
D3OXY
JWT (JSON Web Tokens) have become incredibly popular for authentication in web applications, often marketed as a “stateless” solution that simplifies session management. However, this popularity might be misplaced, and the promise of stateless authentication is largely a myth. Let’s explore why you might want to reconsider using JWTs for authentication.
The main selling point of JWTs is the promise of stateless authentication - the idea that you can verify a user’s identity without storing any session information on the server. However, this promise falls apart when we examine real-world requirements:
// A typical JWT implementation
const token = jwt.sign({ userId: user.id, role: user.role }, process.env.JWT_SECRET, { expiresIn: "24h" });
// Problem: This token can't be revoked if compromised!
Once a JWT is issued, it remains valid until expiration. If compromised, you have limited options:
JWTs are significantly larger than session IDs:
Session ID: "sess:a1b2c3d4" (12 bytes)
JWT: "eyJhbGciOiJIUzI1NiIs..." (800+ bytes)
This extra data is sent with every request, increasing bandwidth usage and parsing overhead.
Here’s why traditional session IDs are often better:
Example of a simple, effective session-based auth:
// Server-side session management
app.post("/login", async (req, res) => {
const user = await authenticate(req.body);
req.session.userId = user.id;
// Session ID is automatically handled by your session middleware
});
// Invalidation is simple
app.post("/logout", (req, res) => {
req.session.destroy();
// Immediate effect, no waiting for expiration
});
JWTs aren’t all bad. They’re useful for:
Most applications require state for:
// You often end up with state anyway
const rateLimit = {
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100, // limit each IP to 100 requests per windowMs
};
// This requires state storage!
app.use(rateLimit);
Instead of JWTs, consider:
app.use(
session({
secret: process.env.SESSION_SECRET,
cookie: {
secure: true,
httpOnly: true,
sameSite: "strict",
},
resave: false,
saveUninitialized: false,
})
);
While JWTs have their place, they’re often misused for session management and authentication. The promise of stateless authentication is largely a myth, and traditional session-based authentication often provides better security, performance, and flexibility.
Remember: Architecture decisions should be based on actual requirements, not buzzwords. Sometimes, the traditional approach is the right one.